Inject Extra Content in Widget
Introduction
There are actually two versions of the Dynamic Widget (the all in one UI). The first is the non embedded version, which by default will show up as a button wherever you want to put it on your site. This button will then open the Dynamic modal.
The second is the embedded widget, which does not involve a button but means you can directly embed the modal somewhere.
We’re explaining this because while you can customise everything about the appearance of the standard widget (as per the tutorial), you can go even further with the embedded widget. Let’s learn how to do that here!
Our goal will be to add extra content to the widget above where the top content is currently:
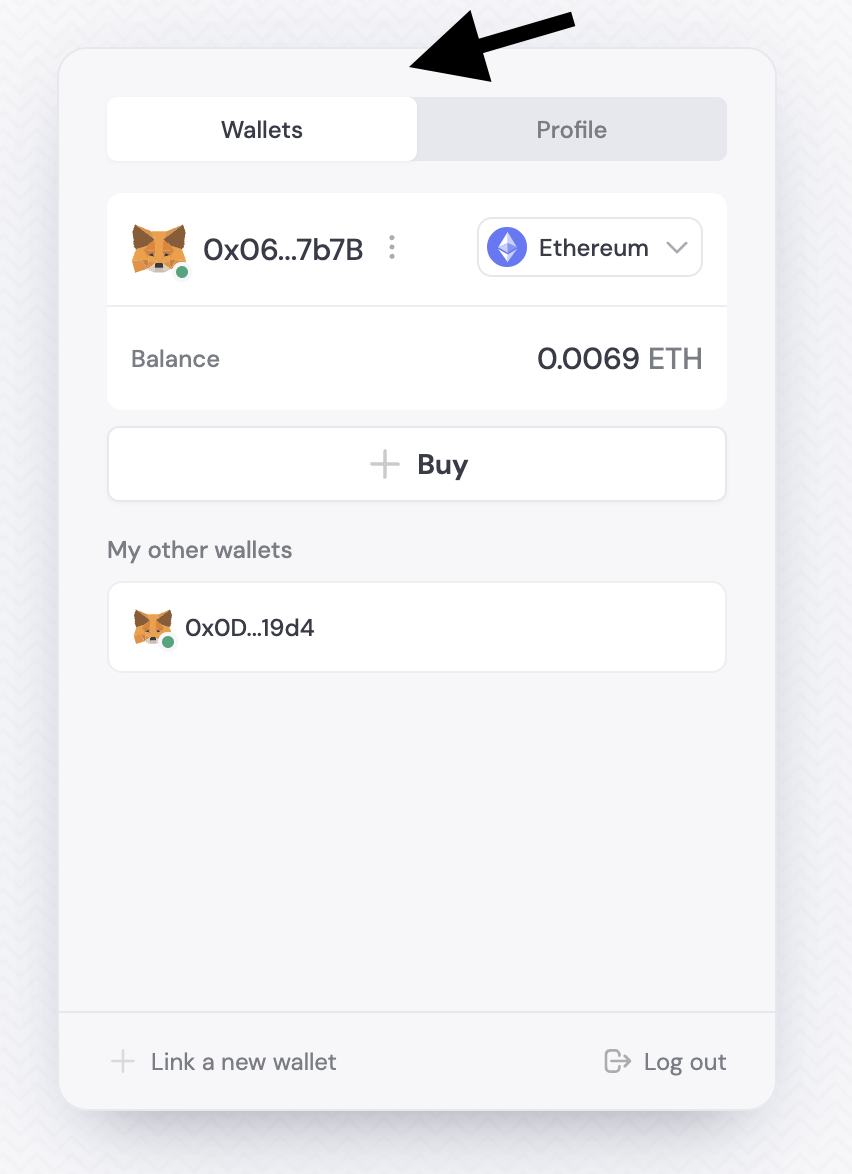
There are two steps:
-
Develop the extra content
-
Add it to the widget
Develop the extra content
The first step is to develop the extra content. This is done by creating a React component that will be rendered inside the widget. This component can be as simple or as complex as you want. For this example, we’ll just add a simple text.
import React from "react";
const ExtraContent = () => (
<div className="extra-content-container">
<p>This is some extra content!</p>
</div>
);
export default ExtraContent;
The one thing we want to make sure of here, is that the styling of the extra content matches the general styling of the widget, so let’s add some css to make sure that happens:
.extra-content-container {
display: flex;
flex-direction: column;
align-items: center;
padding: 1.5rem 1.5rem 0;
}
Add it to the widget
Now we have our content and know that it’s styled to match, we need to add it to the widget. This is done by wrapping the embedded widget in a parent component, and then adding the extra content there. Let’s start by wrapping the widget:
import React from "react";
import { DynamicEmbeddedWidget } from "@dynamic-labs/sdk-react-core";
const WidgetContainer = () => (
<div className="widget-container">
<DynamicEmbeddedWidget />
</div>
);
export default WidgetContainer;
Next we can add the extra content:
import React from "react";
import { DynamicEmbeddedWidget } from "@dynamic-labs/sdk-react-core";
import ExtraContent from "./ExtraContent";
const WidgetContainer = () => (
<div className="widget-container">
<ExtraContent />
<DynamicEmbeddedWidget />
</div>
);
If you run this, you’ll see that it’s working but the extra content shows up on every single state of the modal, and that’s probably not what we want.
Let’s say that we only want this extra content to show up on the profile page after we have logged in.
The best way to check that is with the useIsLoggedIn
hook:
import { useIsLoggedIn } from "@dynamic-labs/sdk-react-core";
const isLoggedIn = useIsLoggedIn();
Now we can use these values to determine whether or not to show the extra content:
import React from "react";
import {
DynamicEmbeddedWidget,
useIsLoggedIn,
} from "@dynamic-labs/sdk-react-core";
import ExtraContent from "./ExtraContent";
const WidgetContainer = () => {
const isLoggedIn = useIsLoggedIn();
return (
<div className="widget-container">
{isLoggedIn && <ExtraContent />}
<DynamicEmbeddedWidget />
</div>
);
};
That’s it! You’ve just added extra content to the embedded widget. You can now add whatever you want to this extra content, and you can also add it to any other state of the modal. For example, you could add it when the user has a username like so:
import React from "react";
import {
DynamicEmbeddedWidget,
useDynamicContext,
userIsLoggedIn,
} from "@dynamic-labs/sdk-react-core";
const WidgetContainer = () => {
const { user } = useDynamicContext();
const isLoggedIn = userIsLoggedIn();
return (
<div className="widget-container">
{isLoggedIn && user?.username && (
<ExtraContent username={user.username} />
)}
<DynamicEmbeddedWidget />
</div>
);
};
The result should look something like this!
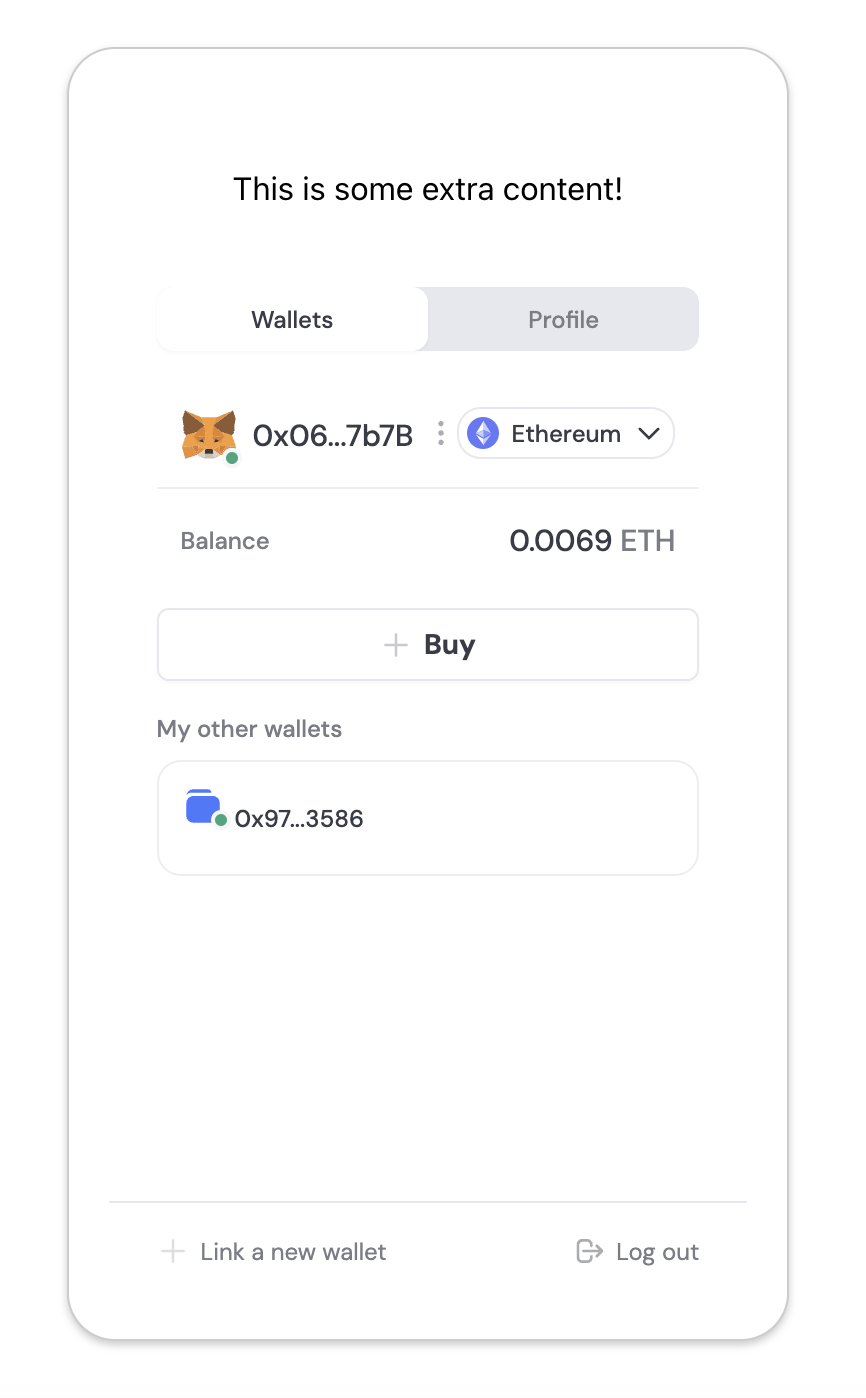
Was this page helpful?